Relearning MSX #30: Complex conditional statements in MSX-C
Posted by Javi Lavandeira in How-to, MSX, Retro, Technology | October 13, 2015In the last post we learnt how to use comparisons in C. This time we’ll see some properties of the comparison operators and also how to test on more complex conditions.
Structure of conditional statements: comparison values
Until now we’ve only seen conditional statements that use the relational operators that we saw in the previous post. However, in adition to these relational operators we can use almost anything as the condition. For example, the code below shows how to test a condition elegantly:
In this example, whether the puts() statement will run or not depends on the value of whatever is inside the parentheses following the if keyword:
- If the value inside the parentheses is zero, the condition is false and the puts() does not run
- If the value inside the parentheses is anything other than zero then the condition is true and the puts() statement runs
Because of this, the code above will not do anything if the variable error contains a zero, and it will print the message “Something bad happened.” if it contains any non-zero value.
We say that C uses the data in the if() statement as the comparison value. This means that when we put an expression inside a conditional statement, C looks at the result of the expression and uses that as the comparison value. A result of zero is treated as false, and any result different from zero is treated as true. This program illustrates this:
Compile and run this program and you’ll get this output:
As you can see, all the conditions are true except for the expression 1 – 1, which gives zero as result.
This also works the other way around: comparison operators give 1 as a result when the comparison is true, and 0 when the comparison is false. For example:
Joining comparisons together: logical operators
If we need to check complex conditions we could nest several if/else statements, but there’s an easier way to check conditions such as A and B or A or B: logical operators.
MSX-BASIC uses the same operators for bitwise and logical operations: AND, OR and NOT. However, the logical operators in C are different from the bitwise arithmetic operators that we saw in a previous post (&, |, ^ and ~). See the following table:
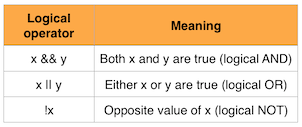
Logical operators in C
Logical AND (&&): Test that both x and y are true
When we form an expression using the && operator, the result of the expression is true only if both operands are true. See this example:
This program reads a letter from the keyboard into the character variable c, then checks that the value in c is higher than or equal to ‘a’ and also (&&) lower than or equal to ‘z’. If both of these conditions are true then the character is lower case.
Logical OR (||):Test whether x is true, or y is true, or both are true
An expression that uses the || operator is true if at least one of its operands is true. For example:
This program prints a message asking for confirmation and then reads a character from the keyboard. If the character is either ‘y’ or ‘Y’ then it takes the reply as a yes, otherwise it takes the reply as a no:
Logical NOT (!): Negates the condition
The last logical operator is very simple. It inverts the logical value of its operand: true becomes false and false becomes true. See this example program:
The condition in this program works like this: if you press any key other than ‘n’ or ‘N’ then the program takes that as a yes, otherwise it handles the key as a no.
Variable assignments in conditional statements
Another interesting characteristic of conditional statements is that we can assign values to variables inside them and use the value assigned as the condition value. This sometimes makes programs a bit more difficult to read, but it’s considered more efficient and elegant. In the end, whether you follow this style or not is up to you.
Look at this example to see how this works:
This program first waits for a key from the keyboard using getche() and assigns the letter read to the variable c. Then it checks the value of c to see if it’s equal to ‘y’. If it is, then it prints “Ok” on the screen.
Remember to put the assignment inside parentheses in order to avoid issues with the priority of operators:
(c = getche())
Summary
We’ve seen how conditionals in C decide whether a condition is true or not, an we’ve learnt that C gives a value of 1 to a true condition, and 0 to a false one. We’ve learnt how to chain comparisons using the logical operators (&&, || and !). We’ve also seen how to assign values to variables inside a condition in order to write more efficient code, perhaps at the expense of readability.
In the next post…
The next post will be lots of fun: loops!
This series of articles is supported by your donations. If you’re willing and able to donate, please visit the link below to register a small pledge. Every little amount helps.
Javi Lavandeira’s Patreon page
Hey, great article again. Small bug in your a-z example (you check between a and a, inclusive :))…
Ooops, thanks for noticing! I’ll fix it later at lunch time!
Fixed. Thanks a lot!